-
Notifications
You must be signed in to change notification settings - Fork 4.2k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
GradientPicker: Auto-generate readme #67250
Changes from all commits
File filter
Filter by extension
Conversations
Jump to
Diff view
Diff view
There are no files selected for viewing
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,110 +1,148 @@ | ||
# GradientPicker | ||
|
||
GradientPicker is a React component that renders a color gradient picker to define a multi step gradient. There's either a _linear_ or a _radial_ type available. | ||
<!-- This file is generated automatically and cannot be edited directly. Make edits via TypeScript types and TSDocs. --> | ||
|
||
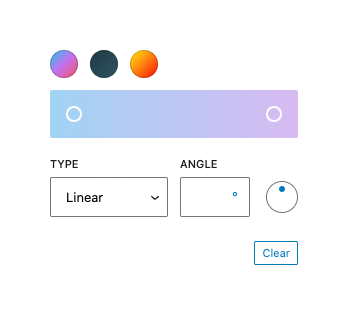 | ||
<p class="callout callout-info">See the <a href="https://wordpress.github.io/gutenberg/?path=/docs/components-gradientpicker--docs">WordPress Storybook</a> for more detailed, interactive documentation.</p> | ||
|
||
## Usage | ||
|
||
Render a GradientPicker. | ||
GradientPicker is a React component that renders a color gradient picker to | ||
define a multi step gradient. There's either a _linear_ or a _radial_ type | ||
available. | ||
|
||
```jsx | ||
import { useState } from 'react'; | ||
import { GradientPicker } from '@wordpress/components'; | ||
|
||
const myGradientPicker = () => { | ||
const [ gradient, setGradient ] = useState( null ); | ||
|
||
return ( | ||
<GradientPicker | ||
value={ gradient } | ||
onChange={ ( currentGradient ) => setGradient( currentGradient ) } | ||
gradients={ [ | ||
{ | ||
name: 'JShine', | ||
gradient: | ||
'linear-gradient(135deg,#12c2e9 0%,#c471ed 50%,#f64f59 100%)', | ||
slug: 'jshine', | ||
}, | ||
{ | ||
name: 'Moonlit Asteroid', | ||
gradient: | ||
'linear-gradient(135deg,#0F2027 0%, #203A43 0%, #2c5364 100%)', | ||
slug: 'moonlit-asteroid', | ||
}, | ||
{ | ||
name: 'Rastafarie', | ||
gradient: | ||
'linear-gradient(135deg,#1E9600 0%, #FFF200 0%, #FF0000 100%)', | ||
slug: 'rastafari', | ||
}, | ||
] } | ||
/> | ||
); | ||
const MyGradientPicker = () => { | ||
const [ gradient, setGradient ] = useState( null ); | ||
|
||
return ( | ||
<GradientPicker | ||
value={ gradient } | ||
onChange={ ( currentGradient ) => setGradient( currentGradient ) } | ||
gradients={ [ | ||
{ | ||
name: 'JShine', | ||
gradient: | ||
'linear-gradient(135deg,#12c2e9 0%,#c471ed 50%,#f64f59 100%)', | ||
slug: 'jshine', | ||
}, | ||
{ | ||
name: 'Moonlit Asteroid', | ||
gradient: | ||
'linear-gradient(135deg,#0F2027 0%, #203A43 0%, #2c5364 100%)', | ||
slug: 'moonlit-asteroid', | ||
}, | ||
{ | ||
name: 'Rastafarie', | ||
gradient: | ||
'linear-gradient(135deg,#1E9600 0%, #FFF200 0%, #FF0000 100%)', | ||
slug: 'rastafari', | ||
}, | ||
] } | ||
/> | ||
); | ||
}; | ||
``` | ||
|
||
## Props | ||
|
||
The component accepts the following props: | ||
### `__experimentalIsRenderedInSidebar` | ||
|
||
### `className`: `string` | ||
Whether this is rendered in the sidebar. | ||
|
||
The class name added to the wrapper. | ||
- Type: `boolean` | ||
- Required: No | ||
- Default: `false` | ||
|
||
- Required: No | ||
### `asButtons` | ||
|
||
### `value`: `string` | ||
Whether the control should present as a set of buttons, | ||
each with its own tab stop. | ||
|
||
The current value of the gradient. Pass a css gradient like `linear-gradient(90deg, rgb(6, 147, 227) 0%, rgb(155, 81, 224) 100%)`. Optionally pass in a `null` value to specify no gradient is currently selected. | ||
- Type: `boolean` | ||
- Required: No | ||
- Default: `false` | ||
|
||
- Required: No | ||
- Default: `linear-gradient(90deg, rgb(6, 147, 227) 0%, rgb(155, 81, 224) 100%)` | ||
### `aria-label` | ||
|
||
### `onChange`: `( currentGradient: string | undefined ) => void` | ||
A label to identify the purpose of the control. | ||
|
||
The function called when a new gradient has been defined. It is passed the `currentGradient` as an argument. | ||
- Type: `string` | ||
- Required: No | ||
|
||
- Required: Yes | ||
### `aria-labelledby` | ||
|
||
### `gradients`: `GradientsProp[]` | ||
An ID of an element to provide a label for the control. | ||
|
||
An array of objects of predefined gradients displayed above the gradient selector. | ||
- Type: `string` | ||
- Required: No | ||
|
||
### `className` | ||
|
||
The class name added to the wrapper. | ||
|
||
- Required: No | ||
- Default: `[]` | ||
- Type: `string` | ||
- Required: No | ||
|
||
### `clearable`: `boolean` | ||
### `clearable` | ||
|
||
Whether the palette should have a clearing button or not. | ||
|
||
- Required: No | ||
- Default: true | ||
- Type: `boolean` | ||
- Required: No | ||
- Default: `true` | ||
|
||
### `disableCustomGradients` | ||
|
||
If true, the gradient picker will not be displayed and only defined | ||
gradients from `gradients` will be shown. | ||
|
||
- Type: `boolean` | ||
- Required: No | ||
- Default: `false` | ||
|
||
### `gradients` | ||
|
||
An array of objects as predefined gradients displayed above the gradient | ||
selector. Alternatively, if there are multiple sets (or 'origins') of | ||
gradients, you can pass an array of objects each with a `name` and a | ||
`gradients` array which will in turn contain the predefined gradient objects. | ||
|
||
- Type: `GradientsProp` | ||
- Required: No | ||
- Default: `[]` | ||
|
||
### `disableCustomGradients`: `boolean` | ||
### `headingLevel` | ||
|
||
If true, the gradient picker will not be displayed and only defined gradients from `gradients` are available. | ||
The heading level. Only applies in cases where gradients are provided | ||
from multiple origins (i.e. when the array passed as the `gradients` prop | ||
contains two or more items). | ||
|
||
- Required: No | ||
- Default: false | ||
- Type: `1 | 2 | 3 | 4 | 5 | 6 | "1" | "2" | "3" | "4" | ...` | ||
- Required: No | ||
- Default: `2` | ||
|
||
### `headingLevel`: `1 | 2 | 3 | 4 | 5 | 6 | '1' | '2' | '3' | '4' | '5' | '6'` | ||
### `loop` | ||
|
||
The heading level. Only applies in cases where gradients are provided from multiple origins (ie. when the array passed as the `gradients` prop contains two or more items). | ||
Prevents keyboard interaction from wrapping around. | ||
Only used when `asButtons` is not true. | ||
|
||
- Required: No | ||
- Default: `2` | ||
- Type: `boolean` | ||
- Required: No | ||
- Default: `true` | ||
|
||
### `asButtons`: `boolean` | ||
### `onChange` | ||
|
||
Whether the control should present as a set of buttons, each with its own tab stop. | ||
The function called when a new gradient has been defined. It is passed to | ||
the `currentGradient` as an argument. | ||
|
||
- Required: No | ||
- Default: `false` | ||
- Type: `(currentGradient: string) => void` | ||
- Required: Yes | ||
|
||
### `loop`: `boolean` | ||
### `value` | ||
|
||
Prevents keyboard interaction from wrapping around. Only used when `asButtons` is not true. | ||
The current value of the gradient. Pass a css gradient string (See default value for example). | ||
Optionally pass in a `null` value to specify no gradient is currently selected. | ||
|
||
- Required: No | ||
- Default: `true` | ||
- Type: `string` | ||
- Required: No | ||
- Default: `'linear-gradient(135deg,rgba(6,147,227,1) 0%,rgb(155,81,224) 100%)'` |
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,5 @@ | ||
{ | ||
"$schema": "../../schemas/docs-manifest.json", | ||
"displayName": "GradientPicker", | ||
"filePath": "./index.tsx" | ||
} |
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -166,44 +166,44 @@ function Component( props: PickerProps< any > ) { | |
} | ||
|
||
/** | ||
* GradientPicker is a React component that renders a color gradient picker to | ||
* GradientPicker is a React component that renders a color gradient picker to | ||
* define a multi step gradient. There's either a _linear_ or a _radial_ type | ||
* available. | ||
* | ||
* ```jsx | ||
*import { GradientPicker } from '@wordpress/components'; | ||
*import { useState } from '@wordpress/element'; | ||
* import { useState } from 'react'; | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. We're switching our docs to import directly from |
||
* import { GradientPicker } from '@wordpress/components'; | ||
* | ||
*const myGradientPicker = () => { | ||
* const [ gradient, setGradient ] = useState( null ); | ||
* const MyGradientPicker = () => { | ||
* const [ gradient, setGradient ] = useState( null ); | ||
* | ||
* return ( | ||
* <GradientPicker | ||
* value={ gradient } | ||
* onChange={ ( currentGradient ) => setGradient( currentGradient ) } | ||
* gradients={ [ | ||
* { | ||
* name: 'JShine', | ||
* gradient: | ||
* 'linear-gradient(135deg,#12c2e9 0%,#c471ed 50%,#f64f59 100%)', | ||
* slug: 'jshine', | ||
* }, | ||
* { | ||
* name: 'Moonlit Asteroid', | ||
* gradient: | ||
* 'linear-gradient(135deg,#0F2027 0%, #203A43 0%, #2c5364 100%)', | ||
* slug: 'moonlit-asteroid', | ||
* }, | ||
* { | ||
* name: 'Rastafarie', | ||
* gradient: | ||
* 'linear-gradient(135deg,#1E9600 0%, #FFF200 0%, #FF0000 100%)', | ||
* slug: 'rastafari', | ||
* }, | ||
* ] } | ||
* /> | ||
* ); | ||
*}; | ||
* return ( | ||
* <GradientPicker | ||
* value={ gradient } | ||
* onChange={ ( currentGradient ) => setGradient( currentGradient ) } | ||
* gradients={ [ | ||
* { | ||
* name: 'JShine', | ||
* gradient: | ||
* 'linear-gradient(135deg,#12c2e9 0%,#c471ed 50%,#f64f59 100%)', | ||
* slug: 'jshine', | ||
* }, | ||
* { | ||
* name: 'Moonlit Asteroid', | ||
* gradient: | ||
* 'linear-gradient(135deg,#0F2027 0%, #203A43 0%, #2c5364 100%)', | ||
* slug: 'moonlit-asteroid', | ||
* }, | ||
* { | ||
* name: 'Rastafarie', | ||
* gradient: | ||
* 'linear-gradient(135deg,#1E9600 0%, #FFF200 0%, #FF0000 100%)', | ||
* slug: 'rastafari', | ||
* }, | ||
* ] } | ||
* /> | ||
* ); | ||
* }; | ||
*``` | ||
* | ||
*/ | ||
|
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -36,7 +36,7 @@ type GradientPickerBaseProps = { | |
clearable?: boolean; | ||
/** | ||
* The heading level. Only applies in cases where gradients are provided | ||
* from multiple origins (ie. when the array passed as the `gradients` prop | ||
* from multiple origins (i.e. when the array passed as the `gradients` prop | ||
* contains two or more items). | ||
* | ||
* @default 2 | ||
|
@@ -58,19 +58,17 @@ type GradientPickerBaseProps = { | |
loop?: boolean; | ||
} & ( | ||
| { | ||
// TODO: [#54055] Either this or `aria-labelledby` should be required | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Moving the todo out of JSDoc. |
||
/** | ||
* A label to identify the purpose of the control. | ||
* | ||
* @todo [#54055] Either this or `aria-labelledby` should be required | ||
*/ | ||
'aria-label'?: string; | ||
'aria-labelledby'?: never; | ||
} | ||
| { | ||
// TODO: [#54055] Either this or `aria-label` should be required | ||
/** | ||
* An ID of an element to provide a label for the control. | ||
* | ||
* @todo [#54055] Either this or `aria-label` should be required | ||
*/ | ||
'aria-labelledby'?: string; | ||
'aria-label'?: never; | ||
|
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Fixed some formatting here.